Getting Started
Quickstart
Connect to QuickBooks Desktop via a modern API in 5 minutes.
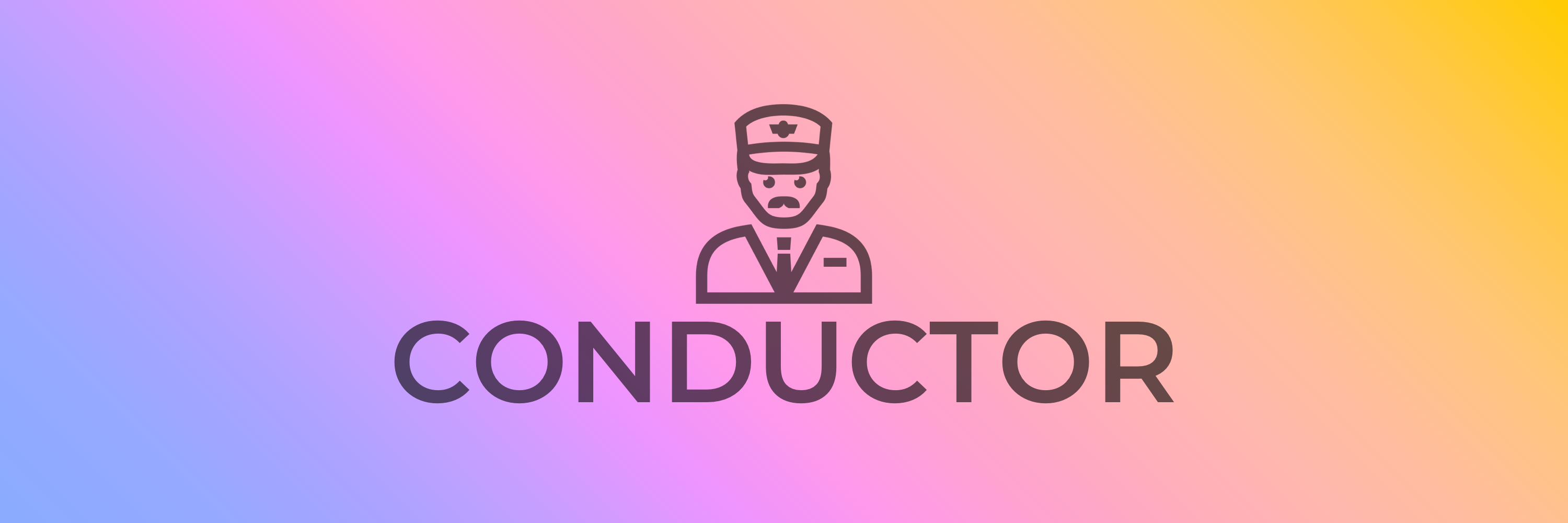
Welcome! 👋 Follow the instructions below to connect your QuickBooks Desktop instance and start working with your data in just a few minutes. You’ll be able to fetch, create, and update any object type in real-time with our modern APIs and SDKs.
Part 1: Set up your Conductor account
Part 2: Connect to QuickBooks Desktop
Important: A QuickBooks Desktop instance for testing is required. If you do not have one, you can create a free test instance.