Quickstart
Create your first EndUser and connect to QuickBooks Desktop in 5 minutes.
The following server-side walkthrough will guide you through connecting a QuickBooks Desktop instance to your Conductor account. In just a few minutes, you’ll be able to create, read, and update data in your QuickBooks Desktop instance.
Requirements
- A Conductor API key pair: one secret key, one publishable key. To obtain these, please join our private beta.
- A QuickBooks Desktop instance for testing. If you do not have one, you can create a free test instance.
- Node.js v16 or later.
Create your first EndUser and IntegrationConnection
Install Conductor for Node.js
Create an EndUser and save its ID to your database
An EndUser is a user of your application for whom we are creating an IntegrationConnection. Each EndUser can represent an individual or an entire company/organization of multiple users within your application. You can attach one or more IntegrationConnections to this EndUser.
After you create your EndUser, you must save the EndUser’s id
to your database for sending requests to their IntegrationConnections in the future.
For example, if your database has a users
table, you can store the id
in a column like conductor_end_user_id
.
Either create the EndUser via the web dashboard or use the following code on your server to create an EndUser programmatically:
This API call will return the newly created EndUser object, for example:
{
// ❗❗ Save this `id` to your database!
id: "end_usr_1234567abcdefg",
objectType: "end_user",
companyName: "Big Construction Co.",
sourceId: "12345678-abcd-abcd-example-1234567890ab",
email: "bob@bigconstruction.com",
createdAt: "2022-11-16 23:51:08.996+00"
}
Create an AuthSession
Before you can read/write data to/from an EndUser’s IntegrationConnection, your EndUser must complete the connection authentication flow. The following operation creates a session and returns a URL to redirect your end-user.
{
id: "int_conn_auth_sess_1234567abcdefg",
endUserId: "end_usr_1234567abcdefg",
clientSecret: "auth_sess_client_secret_1234567abcdefg",
expiresAt: "2022-11-16 23:51:08.996+00",
// 👇 Visit this URL to launch the authentication flow.
authFlowUrl: "https://connect.conductor.is/qbd/auth_sess_client_secret_1234567abcdefg?key={{YOUR_PUBLISHABLE_KEY}}"
}
Complete the authentication flow
Visit the returned authSession.authFlowUrl
in your browser on the same computer or instance as your QuickBooks Desktop installation. This authentication flow will guide you through connecting your QuickBooks Desktop instance to Conductor.
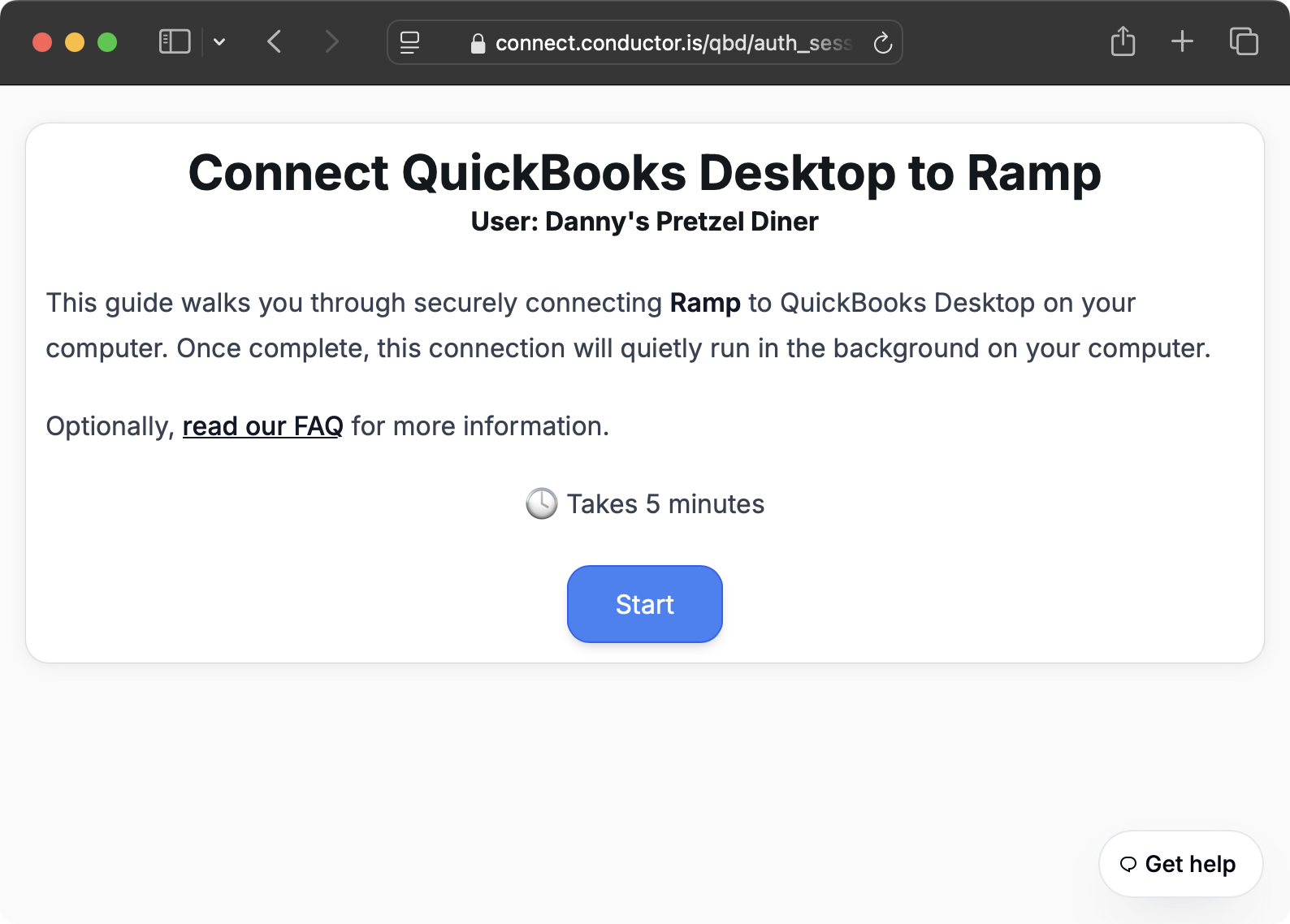
You're done!
After completing the authentication flow, you can access your QuickBooks Desktop instance through the Conductor library. The following example fetches a list of invoices from your QuickBooks Desktop instance.
[
{
ListID: '80000001-1693947446',
TimeCreated: '2023-09-05T13:57:26-07:00',
TimeModified: '2023-09-17T18:13:59-07:00',
EditSequence: 1694999639,
Name: "Annie's Alligators",
FullName: "Annie's Alligators",
IsActive: true,
Sublevel: 0,
Balance: 35693.49,
TotalBalance: 35693.49,
JobStatus: 'None'
},
{...},
{...}
]